This is a step by step guide to help you use The Graph to query Ethereum data in Python. The Graph project is an indexing system for querying decentralized networks. You can use The Graph to query systems like Ethereum, IPFS, etc.
Before we get started lets go over a few definitions:
- GraphQL is a query syntax language
- The Graph is a blockchain project that uses the GraphQL query language. The project allows anyone to build and publish an open API called a subgraph
The Graph (project) uses GraphQL which is a syntax language that describes how to ask for data. This syntax isn’t tied to a specific type of database or storage engine and is instead backed by your existing code and data. GraphQL is about asking for specific fields from an object.
GraphQL
Let’s start by looking at a very simple GraphQL query structure and the results we get when we run it. Once a GraphQL service is running, it can receive GraphQL queries to execute. The service checks the query to ensure it only refers to the types and fields defined, and then runs the function to produce a result.
As an example view the structure of the query below:

The GraphQL query above could produce the results below:
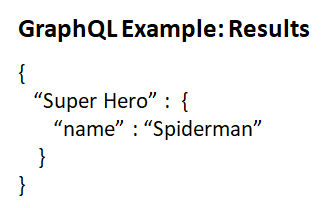
You can see that the query has the same structure as the result. This is essential to GraphQL and as a result the server knows exactly what fields the client is asking for.
Use GraphQL to:
- Search for data
- Pass arguments in your request
- Use aliases
- Fragments and more
Visit GraphQL to learn more about how to write complex GraphQL queries.
The Graph
To get a better understanding of what The Graph project is and how it works visit thegraph.com/docs. It explains how to deploy a subgraph and how to query the subgraph for data. A subgraph defines which data The Graph will index from Ethereum, and how it will store it. Once a subgraph is deployed it can be queried using the GraphQL syntax.
For this tutorial we will focus on querying data from a subgraph.
- Visit The Graph Explorer to view all of the hosted subgraphs that exist for the Ethereum blockchain. Each one of these hosted services (subgraphs) can be queried for data.
- Select a subgraph page and take notice of the http query address and the playground section of the page
- The http query address is needed in your Python code and is the endpoint that contains the blockchain data. This service will execute your GraphQL queries.
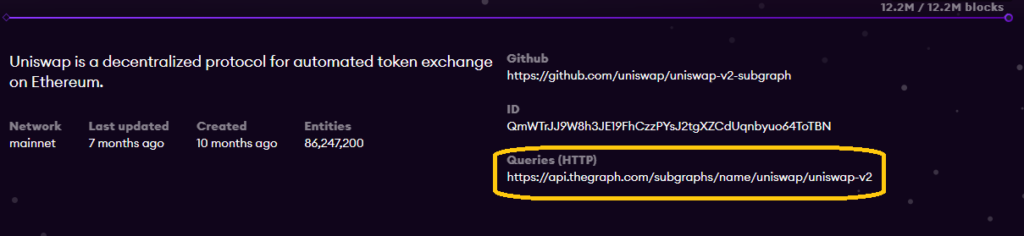
4. Make sure you experiment with the Playground. This section of the website will allow you to construct and test out your GraphQL Ethereum blockchain queries.
- Select a sample query
- The sample query is displayed and can be edited
- Press the run button
- Results are displayed
- The far right side of the screen displays a list of fields that you can add to your query

Using The Graph in Python
Next lets use a few of the queries that we constructed in The Graph’s Playground and use it in our Python code to request different data from the Ethereum blockchain.
The sample Python code below contains a generic function that is used to make a post request to a subgraph. To use different subgraphs you need to change the url end point and GraphQL syntax. I included a print statement and pretty print statement (easier to read) at the end of the program so the results from the Ethereum blockchain will print out in your console.
Example 1: Query Aave on the Ethereum blockchain for a list of the last 10 flash loans by time stamp using GraphQL in Python
import requests
# pretty print is used to print the output in the console in an easy to read format
from pprint import pprint
# function to use requests.post to make an API call to the subgraph url
def run_query(q):
# endpoint where you are making the request
request = requests.post('https://api.thegraph.com/subgraphs/name/aave/protocol'
'',
json={'query': query})
if request.status_code == 200:
return request.json()
else:
raise Exception('Query failed. return code is {}. {}'.format(request.status_code, query))
# The Graph query - Query aave for a list of the last 10 flash loans by time stamp
query = """
{
flashLoans (first: 10, orderBy: timestamp, orderDirection: desc,){
id
reserve {
name
symbol
}
amount
timestamp
}
}
"""
result = run_query(query)
# print the results
print('Print Result - {}'.format(result))
print('#############')
# pretty print the results to make it easier to read
pprint(result)
Example 2: Query Uniswap on the Ethereum blockchain for a list of the top 10 pairs using GraphQL in Python
The query below is a leaderboard for Uniswap that details the top ETH liquidity providers in descending order of ETH deposited. This can help you better analyze user behavior, like keeping track of top market movers and observing the relationship between liquidity providers of ETH vs. other tokens. Other fields that can be queried about users include their address, assets bought and sold historically and total fees paid by that user.
import requests
# pretty print is used to print the output in the console in an easy to read format
from pprint import pprint
# function to use requests.post to make an API call to the subgraph url
def run_query(q):
# endpoint where you are making the request
request = requests.post('https://api.thegraph.com/subgraphs/name/uniswap/uniswap-v2'
'',
json={'query': query})
if request.status_code == 200:
return request.json()
else:
raise Exception('Query failed. return code is {}. {}'.format(request.status_code, query))
# The Graph query - Query Uniswap for a list of the top 10 pairs where the reserve is > 1000000 USD and the volume is >50000 USD
query = """
{
pairs(first: 10, where: {reserveUSD_gt: "1000000", volumeUSD_gt: "50000"}, orderBy: reserveUSD, orderDirection: desc) {
id
token0 {
id
symbol
}
token1 {
id
symbol
}
reserveUSD
volumeUSD
}
}
"""
result = run_query(query)
# print the results
print('Print Result - {}'.format(result))
print('#############')
# pretty print the results
pprint(result)
Using The Graph to query Ethereum data in Python is powerful. There is a lot of data that can be queried for report generation and analysis.
This code is for learning and entertainment purposes only. The code has not been audited and use at your own risk. Remember smart contracts are experimental and could contain bugs.