Do you what to create a deflationary token, or a token with a tax or have your token contribute to a developer fund? These are just a few of the ideas that you can implement with a Solidity smart contract. In this tutorial I will explain how to send a percentage of tokens to a specific wallet or burn address when a transfer occurs. We will review two different use cases:
- User sends funds to a smart contract and a percent of the tokens are sent to another address.
- User initiates a token transfer between two parties and a percent of the tokens transferred are sent to another address.
Send a percent of tokens to another address when sending to a smart contract
In the first use case a user sends tokens to a Solidity smart contract. When the smart contract receives the tokens another transfer for a percentage of the tokens is initiated.

In the example above, the smart contract that received Ether sent a percentage to a target address (X wallet). The target address can be:
- a user address
- a smart contract address
- a burn address
- etc.
The code below contains is a modified fallback function that sends 1% of all incoming tokens to another address. Read the comments in the code to understand how this works.
This code is a sample for learning and has not been audited. Use at your own risk.
pragma solidity ^0.5.0;
contract ReceiveETHERandSendPercentageToAnotherAddress{
// if funds are received in this contract then
// Pay 1% to the target address
address payable target = ENTERANADDRESSHERE;
// Fallback function for incoming ether
function () payable external{
//Send 1% to the target address configured above
target.transfer(msg.value/100);
//continue processing
}
}
Try it in Remix
Send a percentage of all token transfers to another address
In the second example you are the owner of a token and have modified the transfer function in the contract. With every transfer a percentage of tokens will be forwarded to a target address.
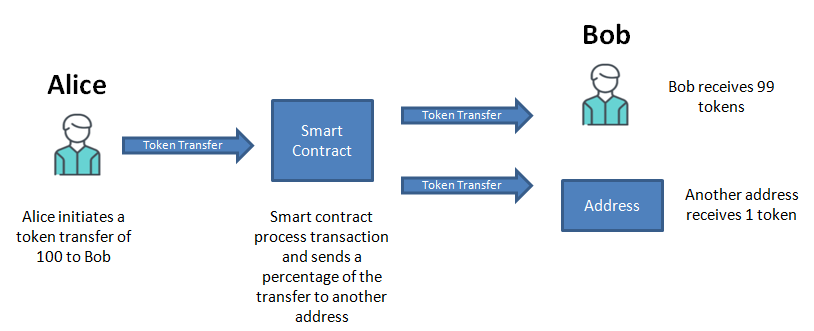
The target address can be:
- a user address
- a smart contract address
- a burn address.
- etc.
The code below contains a modified transfer function (ERC20, ERC721, etc.) that sends 1% of all transfers to another address. Read the comments in the code to understand how this works.
This code is a sample for learning and has not been audited. Use at your own risk.
pragma solidity ^0.6.0;
contract TransfertTokenAndPercentageToTargetAddress{
// pay 1% of all transactions to target address
address payable target = ENTERANADDRESSHERE;
// state variables for your token to track balances and to test
mapping (address => uint) public balanceOf;
uint public totalSupply;
// create a token and assign all the tokens to the creator to test
constructor(uint _totalSupply) public {
totalSupply = _totalSupply;
balanceOf[msg.sender] = totalSupply;
}
// the token transfer function with the addition of a 1% share that
// goes to the target address specified above
function transfer(address _to, uint amount) public {
// calculate the share of tokens for your target address
uint shareForX = amount/100;
// save the previous balance of the sender for later assertion
// verify that all works as intended
uint senderBalance = balanceOf[msg.sender];
// check the sender actually has enough tokens to transfer with function
// modifier
require(senderBalance >= amount, 'Not enough balance');
// reduce senders balance first to prevent the sender from sending more
// than he owns by submitting multiple transactions
balanceOf[msg.sender] -= amount;
// store the previous balance of the receiver for later assertion
// verify that all works as intended
uint receiverBalance = balanceOf[_to];
// add the amount of tokens to the receiver but deduct the share for the
// target address
balanceOf[_to] += amount-shareForX;
// add the share to the target address
balanceOf[target] += shareForX;
// check that everything works as intended, specifically checking that
// the sum of tokens in all accounts is the same before and after
// the transaction.
assert(balanceOf[msg.sender] + balanceOf[_to] + shareForX ==
senderBalance + receiverBalance);
}
}
Try it in Remix
The concept of sending a percentage of tokens to another address during a transfer has a lot of possibilities. Some example uses cases are:
- Burn tokens when a transfer occurs to reduce the token supply
- Send tokens to another address for savings
- Send tokens to a smart contract that redistributes a percentage to another account
- etc.
This code is for learning and entertainment purposes only. The code has not been audited and use at your own risk. Remember smart contracts are experimental and could contain bugs.
Click here for more information about how to use the Ethereum test network and how to obtain test ETH.
Resources
Blockchain Networks
Below is a list of EVM compatible Mainnet and Testnet blockchain networks. Each link contains network configuration, links to multiple faucets for test ETH and tokens, bridge details, and technical resources for each blockchain. Basically everything you need to test and deploy smart contracts or decentralized applications on each chain. For a list of popular Ethereum forums and chat applications click here.
![]() | Ethereum test network configuration and test ETH faucet information |
![]() | Optimistic Ethereum Mainnet and Testnet configuration, bridge details, etc. |
![]() | Polygon network Mainnet and Testnet configuration, faucets for test MATIC tokens, bridge details, etc. |
![]() | Binance Smart Chain Mainnet and Testnet configuration, faucets for test BNB tokens, bridge details, etc. |
![]() | Fanton networt Mainnet and Testnet configuration, faucets for test FTM tokens, bridge details, etc. |
![]() | Kucoin Chain Mainnet and Testnet configuration, faucets for test KCS tokens, bridge details, etc. |
Web3 Software Libraries
You can use the following libraries to interact with an EVM compatible blockchain.
- Python: Web3.py Python library for interacting with Ethereum. Web3.py examples
- Js: web3.js Ethereum JavaScript API
- Java: web3j Web3 Java Ethereum Ðapp API
- PHP: web3.php A php interface for interacting with the Ethereum blockchain and ecosystem.
Nodes
Learn how to run a Geth node. Read getting started with Geth to run an Ethereum node.
Fix a transaction
How to fix a pending transaction stuck on Ethereum or EVM compatible chain