Below are instructions to deploy a Solidity smart contract to the Polygon / Matic network. The Polygon Network is a layer two solution that is compatible with the Ethereum Virtual Machine. This means you can deploy an Ethereum smart contract to the Polygon Network. Polygon supports Ethereum consensus, smart contracts and staking (instead of POW) for the native Matic token.
For more information about Polygon click here.
Polygon Development Tools
As a Polygon developer you can use the same development tools that are used for development on Ethereum. You can use Remix, Truffle, MetaMask, etc. to develop and interact on the Polygon layer 2 blockchain. Check here for a list of Ethereum developer tools.
Configure MetaMask for the Polygon Test Network
The name of the Polygon test network is Mumbai and below I will explain how to configure your MetaMask wallet to connect to the Polygon Mumbai test network.
Open MetaMask and select:
- Settings
- Network
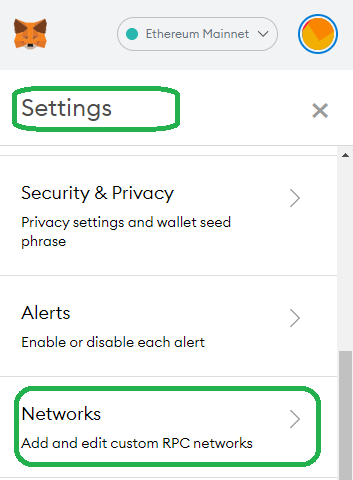
- Select Add Network

- Copy and past the information below in a text editor. It will make it easier to put this information into MetaMask and will prevent the screen from closing on you.
- Type the below information into MetaMask:
- Put in a Network name – “Matic Mumbai Testnet”
- In URL field you can add the URL as “https://rpc-mumbai.matic.today”
- Enter the Chain ID: 80001
- (Optional Fields) Symbol: “maticmum” and Block Explorer URL: “https://mumbai-explorer.matic.today/”
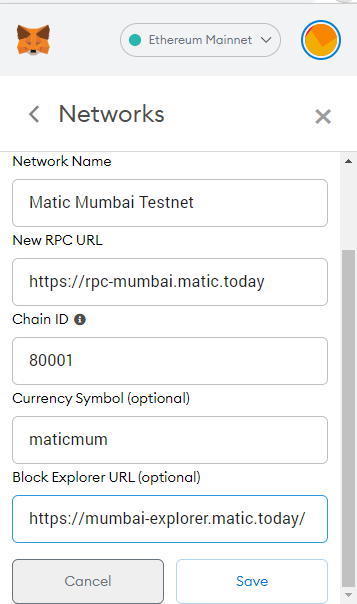
You are done. Now your MetaMask wallet is connected to the Polygon Mumbai test network.
Polygon Faucet for Test Tokens
Now go to the Polygon / Matic faucet and request test MATIC tokens. The tokens are sent to your wallet within seconds. You need to wait 60 seconds to request tokens again.
- Test LINK tokens are available from the Link community faucet.
- Various test tokens can be obtained from Aave’s Mumbai’s test environment.
Now that you are connected to the Polygon network, your wallet is setup, and you have Matic test tokens try to deploy a smart contract.
Deploy Smart Contract to Polygon
You can use Remix to write Solidity smart contracts and deploy them to the Polygon test network. To test this you can copy the Solidity smart contract code below and give it a try.
//define which compiler to use
pragma solidity ^0.5.0;
//contract name is MyFirstPolygonContract
contract MyFirstPolygonContract {
string private name;
uint private amount;
//set
function setName(string memory newName) public {
name = newName;
}
//get
function getName () public view returns (string memory) {
return name;
}
//set
function setAmount(uint newAmount) public {
amount = newAmount;
}
//get
function getAmount() public view returns (uint) {
return amount;
}
}
Try it in Remix
Then submit your transaction to the network in Remix. MetaMask will display Matic Mumbai Testnet in the upper right corner.

To see the transaction confirm on the Polygon network use their block explorer for the test environment.
Below is the Solidity smart contract Polygon transaction that I submitted to the test network. To view the contract click on the newly created contract account number on the top left of the screen (the black line in the picture below is blocking the contract account number).
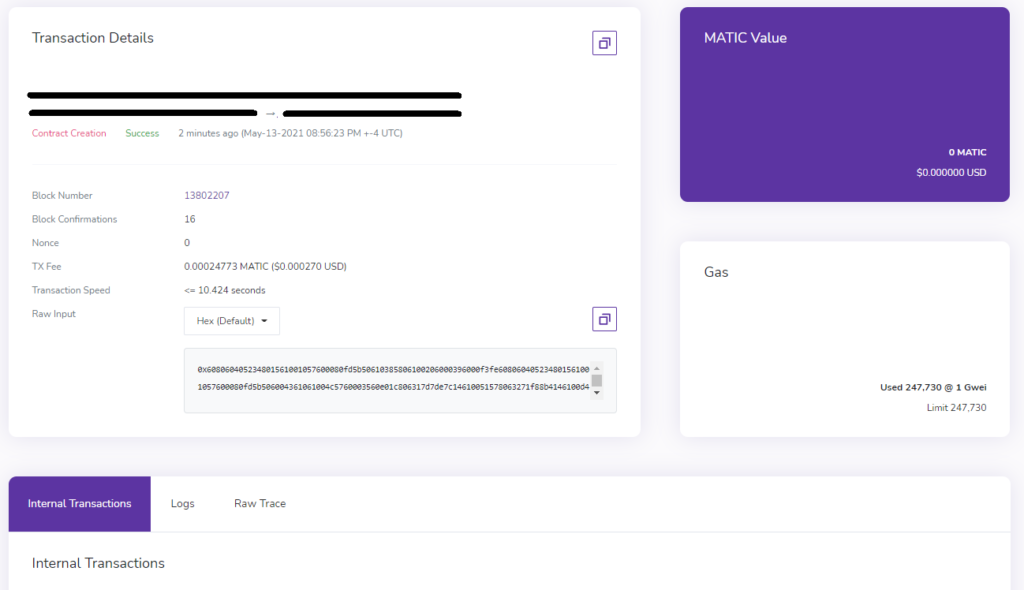
To verify the contract code click on the “Code” menu item in the middle of the screen.

Finally click on the verify and publish button to verify your contract on the network.
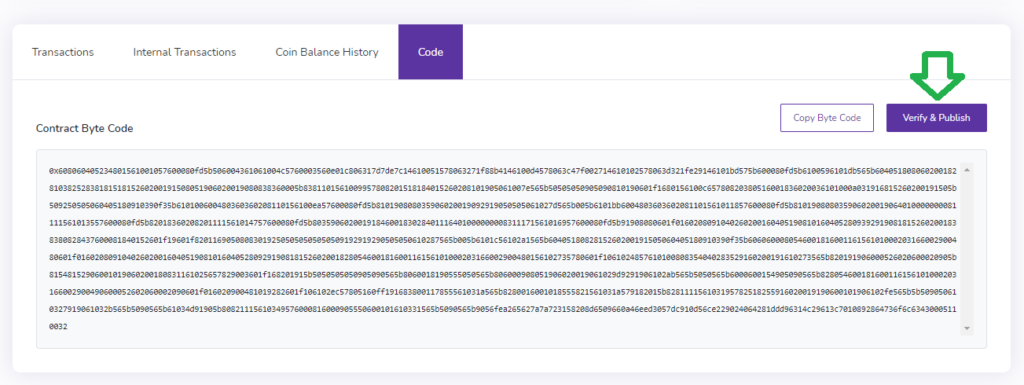
This was a simple process that was very cheap and fast to complete.
Configure MetaMask for the Polygon Mainnet network
The Polygon Mainnet can be configured using the settings below. Copy and past the information below in a text editor.
- It will make it easier to put this into MetaMask and will prevent the screen from closing on you.
- Type the below information into MetaMask by adding a new custom RPC. Follow the instructions above for the test network and use the setting below for the Mainnet.
- Network Name: Matic Mainnet
- New RPC URL: https://rpc-mainnet.maticvigil.com/
- ChainID: 137
- Symbol: MATIC
- Block Explorer URL: https://polygonscan.com/
Transfer Funds from Ethereum to Polygon on the Mainnet
If you have tokens on Ethereum and you want to transfer them to Polygon / Matic you can use the Polygon / Matic Bridge. This bridge is a trustless two way transaction channel and you can read more about it here. To use the bridge visit https://wallet.matic.network/bridge/. It takes approximately 12-15 mins for funds to transfer.
- Navigate to the Polygon / Matic bridge website https://wallet.matic.network/bridge/
- Connect your wallet
- Select the token on the Ethereum chain that you want to move to the Polygon / Matic chain
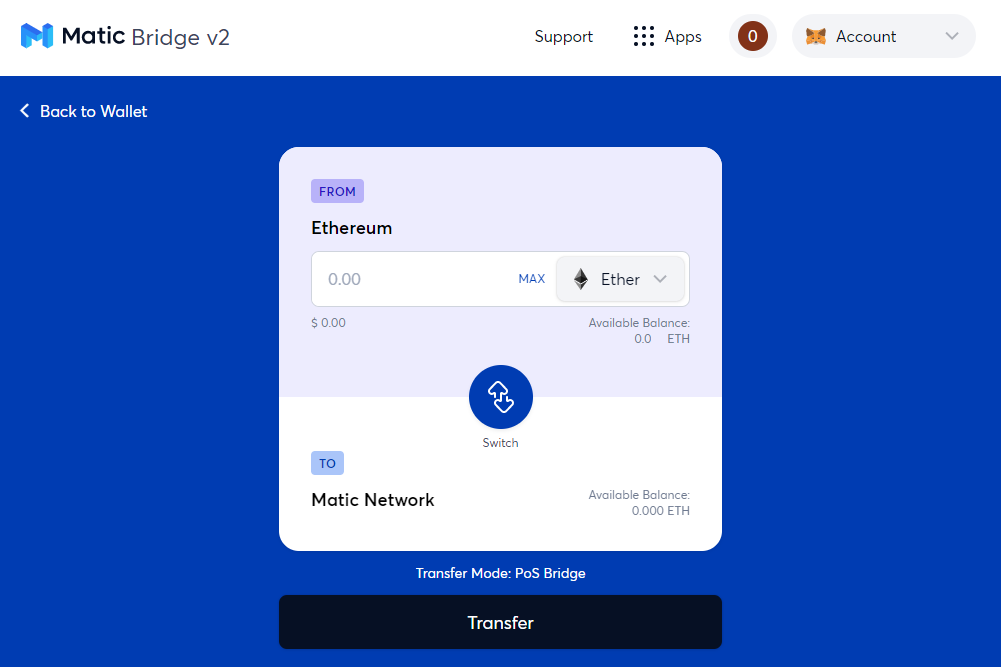
4. After you approve the transfer the process will begin to move funds.
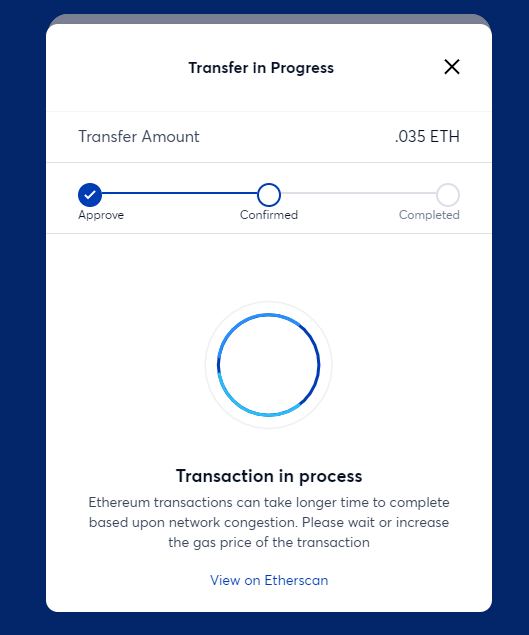
5. After the transaction confirms on the Ethereum network the deposit will finalize on the Polygon / Matic network.
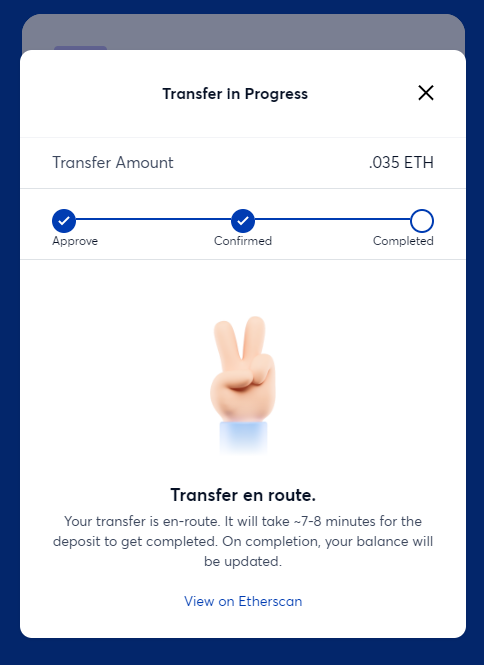
6. You will receive notification that the transaction is complete.
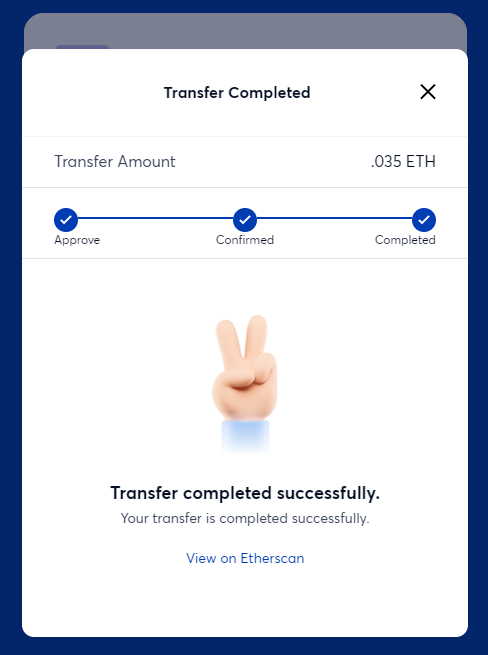
7. Go view your wallet on the Matic block Explorer
8. Select Tokens on the Matic block Explorer and you will see that your ETH or token transfered as an ERC20 token.
9. Copy the ERC20 address and it to MetaMask so you can monitor your token balance.

10. Gas fees on Polygon are very cheap. If needed this site is helpful to swap some funds for a few Matic tokens to use for gas. https://wallet.polygon.technology/gas-swap/. Another option is to go to Quick Swap and transfer your ETH to the native Matic token.
Note – If needed the Quick Swap source code, router address and factor address can be found on Github.
Mapping tokens between Ethereum and Polygon
If you have a token on the Ethereum network and you want to map it to the Polygon network you need to complete this form to enable transfers. https://mapper.matic.today/
Resources
Blockchain Networks
Below is a list of EVM compatible Mainnet and Testnet blockchain networks. Each link contains network configuration, links to multiple faucets for test ETH and tokens, bridge details, and technical resources for each blockchain. Basically everything you need to test and deploy smart contracts or decentralized applications on each chain. For a list of popular Ethereum forums and chat applications click here.
![]() | Ethereum test network configuration and test ETH faucet information |
![]() | Optimistic Ethereum Mainnet and Testnet configuration, bridge details, etc. |
![]() | Polygon network Mainnet and Testnet configuration, faucets for test MATIC tokens, bridge details, etc. |
![]() | Binance Smart Chain Mainnet and Testnet configuration, faucets for test BNB tokens, bridge details, etc. |
![]() | Fanton networt Mainnet and Testnet configuration, faucets for test FTM tokens, bridge details, etc. |
![]() | Kucoin Chain Mainnet and Testnet configuration, faucets for test KCS tokens, bridge details, etc. |
Web3 Software Libraries
You can use the following libraries to interact with an EVM compatible blockchain.
- Python: Web3.py Python library for interacting with Ethereum. Web3.py examples
- Js: web3.js Ethereum JavaScript API
- Java: web3j Web3 Java Ethereum Ðapp API
- PHP: web3.php A php interface for interacting with the Ethereum blockchain and ecosystem.
11 thoughts on “Deploy a smart contract to the Polygon Network”