The Kucoin Chain is a blockchain that uses the Ethereum Virtual Machine (EVM) which means it is Solidity compatible. Below are instructions about how to deploy a Solidity smart contract to the Kucoin Chain “KCC”. The process to deploy a smart contract outlined below is almost identical to other EVM compatible blockchains.
What is the Kucoin Chain
The Kucoin Chain “KCC” claims to be a decentralized public chain with high performance. The chains goals is to solve low transaction performance and high transaction costs seen on other public chains. It is fully compatible with Ethereum and ERC smart contract standards. Kucoin Token “KCS” is the fuel and native token for KCC and is used to pay gas fees. Blocks are produced every 3 seconds for accelerated transaction confirmations and higher chain performance. It has adopted the consensus algorithm of Proof of Staked Authority “PoSA”, which is thought to be more efficient and secure. In all this chain seems to have the same business model as the Binance Smart Chain.
For more information about Kucoin Chain visit their website.
Smart contract Kucoin Chain development tools
As a KCC developer you can use the same development tools that are used for development on Ethereum. You can use Remix, Truffle, MetaMask, etc. to develop on the KCC blockchain. Check here for a list of Ethereum developer tools.
Configure MetaMask for the KCC test network
You can use any Ethereum compatible wallet to configure the KCC network. Some example wallets to use are MetaMask, myetherwallet, imtoken, TokenPocket, etc. Below we will configure MetaMask to connect to KCC.
The name of the KCC test network is KCC-TESTNET and below I will explain how to configure your MetaMask wallet to connect to the Kucoin testnet.
Open MetaMask and select:
- Settings
- Network
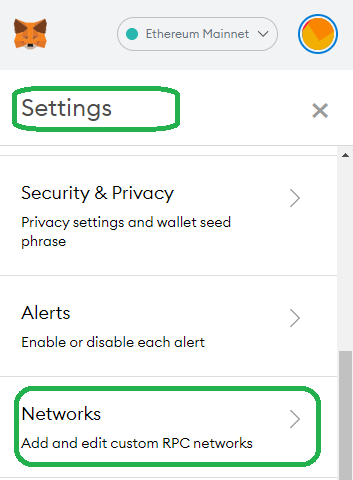
Select Add Network

- Copy and past the information below in a text editor. It will make it easier to put this information into MetaMask and will prevent the screen from closing on you.
- Type the below information into MetaMask:
- Network Name: KCC-TESTNET
- New RPC Url: https://rpc-testnet.kcc.network
- ChainID: 322
- Symbol: KCS
- Block Explorer URL: https://scan-testnet.kcc.network
- WebSocket RPC URL: wss://rpc-ws-testnet.kcc.network
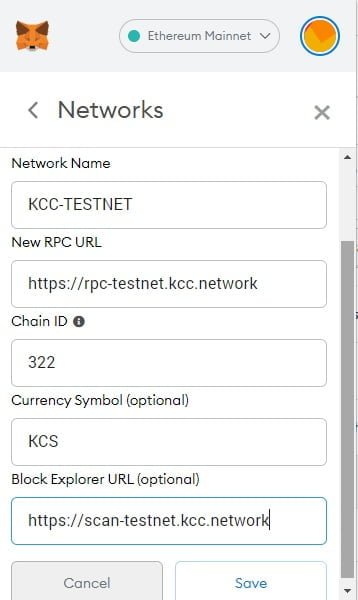
Now your MetaMask wallet is connected to the KCC-TESTNET.
Kucoin faucet for test tokens
Now go to the Kucoin Chain faucet and request test KCS tokens. The tokens are sent to your wallet immediately.
Now that you are connected to the Kucoin testnet, your wallet is setup, and you have KCS test tokens try to deploy a smart contract to the test environment.
Deploy a smart contract to the Kucoin Chain
You can use Remix to write Solidity smart contracts and deploy them to the Kucoin Chain (KCC-TESTNET or KCC-MAINNET). To test this you can copy the Solidity smart contract code below and deploy it to the Kucoin Chain.
// SPDX-License-Identifier: MIT
pragma solidity ^0.6.2;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
//simple ERC20 contract to experiment with
//all tokens are assigned to the creator
contract SimpleToken is ERC20 {
//set the name, symbol, and supply at time of deployment in the constructor
//all tokens are assigned to the creator
constructor(
string memory name,
string memory symbol,
uint256 initialSupply
) public ERC20(name, symbol) {
_mint(msg.sender, initialSupply);
}
}
Try it in Remix
Then submit your transaction to the network in Remix. MetaMask will display KCC-TESTNET in the upper right corner.
To see the transaction confirm on the KCC-TESTNET use their block explorer for the test environment.
Use their block explorer to navigate to your smart contract that was submitted to the test network. To view the contract click on the newly created contract account number on the top of the screen (the black line in the picture below is blocking the contract account numbers).
To verify the contract code click on the “Verify and Publish” link at the bottom of the screen. The contract verify and publish process is very similar to Ethereum.

Configure MetaMask for the KCC Mainnet
The KCC Mainnet can be configured using the settings below. Copy and past the information below in a text editor.
- It will make it easier to put this into MetaMask and will prevent the screen from closing on you.
- Type the below information into MetaMask by adding a new custom RPC. Follow the instructions above for the test network and use the setting below for the Mainnet.
- Network Name: KCC-MAINNET
- New RPC Url: https://rpc-mainnet.kcc.network
- ChainID:
321
- Symbol: KCS
- Block Explorer URL: https://explorer.kcc.io/en
- WebSocket RPC URL: wss://rpc-ws-mainnet.kcc.network
Transfer funds from Ethereum to Kucoin Chain on the Mainnet
If you have tokens on Ethereum and you want to transfer them to Kucoin Chain you can use KCC bridge or the AnySwap bridge. These bridges are trustless two way transaction channels and you can read more about them here. It takes approximately 12-15 mins for funds to transfer.
- Navigate to the Anyswap bridge website
- Connect your wallet
- Select the token on the Ethereum chain that you want to move to Kucoin chain.

How to stake KSC on Kucoin Chain
Stake
Call the stake method in the validator smart contract to stake with any validator. The minimum staking amount for each validator is 32 KCS tokens.
Unstake
If you want to unstake your KCS tokens call the unstake method in the validator contract, and wait for 86400 blocks (3 days), then call the withdrawStaking method in the validator contract to withdraw funds.
Run a Kucoin validator node
KCC has a PoSA consensus mechanism which features low transaction costs and fast transactions throughput. PoSA is a combination of PoA and PoS and supports up to 29 validators.
To become a validator you need to create a node then submit a proposal for the active validators to vote on. If more then half of the active validators vote yes you will be eligible to become a validator.
Then user’s can stake their coins to an address that has qualified to become a validator. The minimum amount to stake is 32 KCS. Once the validator’s staking volume ranks in the top 29 it will become an active validator in the next epoch.
All active validators are ordered according to a set of predefined rules. Each validator takes a turn to mine blocks on the chain. If a validator fails to mine a block on time during their round, the active validators who have not been involved in the past n/2 (n is the number of active validators) blocks will randomly perform the block-out. At least n/2+1 active validators work properly to ensure the proper operation of the blockchain.
Resources
Blockchain Networks
Below is a list of EVM compatible Mainnet and Testnet blockchain networks. Each link contains network configuration, links to multiple faucets for test ETH and tokens, bridge details, and technical resources for each blockchain. Basically everything you need to test and deploy smart contracts or decentralized applications on each chain. For a list of popular Ethereum forums and chat applications click here.
![]() | Ethereum test network configuration and test ETH faucet information |
![]() | Optimistic Ethereum Mainnet and Testnet configuration, bridge details, etc. |
![]() | Polygon network Mainnet and Testnet configuration, faucets for test MATIC tokens, bridge details, etc. |
![]() | Binance Smart Chain Mainnet and Testnet configuration, faucets for test BNB tokens, bridge details, etc. |
![]() | Fanton networt Mainnet and Testnet configuration, faucets for test FTM tokens, bridge details, etc. |
![]() | Kucoin Chain Mainnet and Testnet configuration, faucets for test KCS tokens, bridge details, etc. |
Web3 Software Libraries
You can use the following libraries to interact with an EVM compatible blockchain.
- Python: Web3.py Python library for interacting with Ethereum. Web3.py examples
- Js: web3.js Ethereum JavaScript API
- Java: web3j Web3 Java Ethereum Ðapp API
- PHP: web3.php A php interface for interacting with the Ethereum blockchain and ecosystem.
Nodes
Learn how to run a Geth node. Read getting started with Geth to run an Ethereum node.
Fix a transaction
How to fix a pending transaction stuck on Ethereum or EVM compatible chain