A function is a block of organized and reusable code that performs an action. Functions allow a programmer to manage code that is modular and reusable. Different programming languages name these blocks of organized code functions, methods, sub-routines, procedures, etc. If you come across these terms just remember that it is the same concept.
Solidity Functions
A function in Solidity is a piece of reusable code which can be called within or outside of a smart contract. As an example:
- a function can call another function in the same contract
- a function can call another function in a different contract
Creating a function eliminates the need of writing the same code multiple times which reduces the chances of a mistake and promotes code organization. The basic break down of a function in Solidity is as follows:
function name(uint x, uint y) public view returns (uint, unit) {}
This function can look overwhelming but it is really easy to understand once you learn the format. Lets break down this function into smaller pieces so we understand its components.
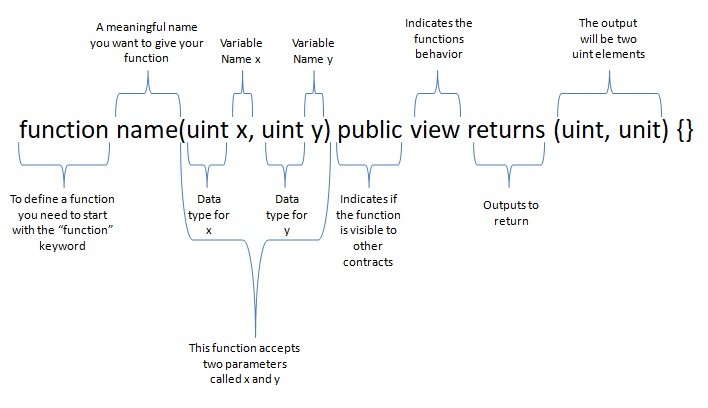
Components of a Solidity function
Each component of the function is described below in more detail. Further explanations with come in future sections. It is important to understand the layout of a solidity function.
Component | Description |
---|---|
function | To define a function you need to start with the function key word. This tells solidity where the function begins |
name | Is a meaningful name that you want to call your function. Keep in mind that you might use this name in other parts of your contract |
uint x, uinty | The data type and variable name. These are parameters you are going to pass into your function to be evaluated or produce some result |
public | Indicates if the function is visibility to other contracts |
view | Indicates the functions behavior. As an example is the function going to only produce a value for view or is it going to save data and modify the state of the block chain |
returns | Outputs to return after executing the function. Cannot be a map or multi dimensional array |
Types of Solidity functions
There are 2 types of functions that you can create in Solidity
- Functions that create transaction on block chain
- Functions that do not create transaction on block chain (view and pure)
Try creating the sample function below in Remix
//define which compiler to use
pragma solidity ^0.5.0;
//contract name is MyFirstContract
contract MyFirstContract {
//create a string state variable called name
string private name;
//use the setName function to set a name
function setName(string memory newName) public {
name = newName;
}
//use the getName function to get the name you set
function getName () public view returns (string memory) {
return name;
}
}
Try it in Remix
Click here for more information about how to use the Ethereum test network and how to obtain test ETH.