In Solidity Gas is a fee which is required to conduct a transaction on the Ethereum blockchain. Gas prices are specified in gwei. Gwei is a denomination of the cryptocurrency Ether. Instead of saying that your gas costs 0.000000001 Ether, you can say your gas costs 1 gwei. Gas is used to allocate resources of the Ethereum virtual machine (EVM) so that wallet transactions and smart contract transactions can self-execute.
Supply and demand on the block chain determine the price of gas in Solidity. If your gas price is too low then your transaction will not execute. Your transaction would be a candidate for the next block. If your gas price is too high then you are over paying.
Simply put gas is a unit of computation. Used to limit computations from running forever and spamming the network. The purpose of gas = to limit the amount of transactions the system will perform.
How is gas in Solidity calculated?
Transaction fee’s are calculated using the following formula = gas used * gas price
There are two components to gas in Solidity and they are important to understand.
- Gas price = How much you are willing to pay for 1 gas. Think of gas price as the amount you pay for 1 gallon or liter of gas at the gas station.
- Lower = slower wait time to process in block
- Higher = faster time to process in block
- Gas Limit = Maximum gas you are willing to use for this transaction. Think of gas limit as the maximum amount of gas your car’s gas tank can hold and you are willing to use for one car trip (with no stops).
- Lower limit – less computations your transaction can process
- Higher limit – the more computations your transaction can process
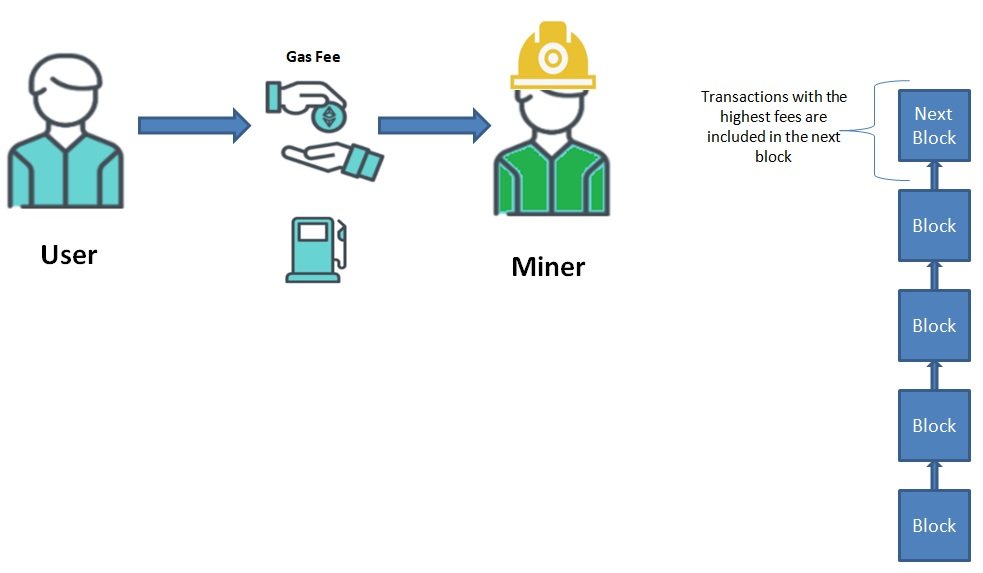
If gas prices are low and your contract does not require a lot of computation to execute a function then your transaction cost will be low. If gas prices are high and you have a complex transaction to execute your transaction cost will be high. This is a variable system that depends on supply and demand of the system and the complexity of the function(s) to execute.
Not all functions require a gas fee to execute. Only transactions to write to the block chain require gas. If you are calling a contract to view data (e.g. a balance, variables in a contract, requesting market data from a decentralized exchange) you DO NOT have to pay a gas fee.
Visit the ETH Gas Station to see the current gas prices in the Ethereum network. The ETH Gas Station’s goal is to increase the transparency of gas prices and Ethereum transaction confirmation times on the Ethereum network.
How to reduce your smart contract gas fees
Blockchain data is replicated on thousands of computer disks around the world. This readily available data makes storage expensive. To reduce your smart contract gas fees reduce your storage. The EVM has two intentionally expensive opcodes:
- SSTORE – Stores data in a storage slot
- SLOAD – Reads data from a storage slot
Below are several helpful tips to reduce smart contract gas costs. You need to perform trial and error to determine the cheapest gas route.
Do not store data on the blockchain if you do not have to
Changing the state of a variable cost gas and is expensive. If you are never going to use the data again do not store it on the blockchain. Ask yourself if you should emit an event instead of using blockchain storage. It is good practice to emit events when storage or state changes in your contract.
Use constant & immutable if you can
For storage variables that do not change use the constant and immutable keywords. Using constant and immutable keywords will reduce your gas cost when SLOAD is called. Immutable means we can set the variable in the constructor but it can never change again. Constant is similar but specified in the declaration and can never changed again. For example:
// use immutable in your variable if it is not going to change
// this will reduce gas costs
address public immutable WETH;
constructor (address wethToken) {
WETH = wethToken;
}
// or use a constant specified in the declaration
address public constant WETH = 0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2
Reduce reads and writes
Reduce the amount of times your smart contract functions access storage. In your function read storage into a memory variable in the beginning of the function and set the storage at the end of the function. This concept of using cold storage and making it warm (using local memory) then saving the data and making it cold again cost less gas.
// accessing storage multiple times costs more gas
function foo () public returns (uint) {
require read storage
require read storage
if statement read storage
else if read storage
set storage
// accessing storage less cost less gas
// read storage once and save it into local memory in your function
// then set storage at the end of your function
function foo () public returns (uint) {
memory = read storage
require memory
require memory
if statement memory
else if memory
set storage
Reduce data type sizes and reorder your structs
Structs are records with more then one data point. The EVM takes each data point and puts them into slots (in order of declaration / top to bottom). Storage slots are 32 bytes each and storage slots are expensive. So resize your data types. For example if you do not need a uint256 type which is 32 bytes make it smaller. For example use:
- uint128 – 16 bytes
- uint96 – 12 bytes
- uint64 – 8 bytes
Then think about the order in which you declare data points in your structs. If each memory slot is 32 bytes order your data points in your structs to fill each slot accordingly. The point is to be conscious of the space you are allocating and using for data in your Solidity smart contract. If you optimize your types and sizes you can save on gas.
Resources
Blockchain Networks
Below is a list of EVM compatible Mainnet and Testnet blockchain networks. Each link contains network configuration, links to multiple faucets for test ETH and tokens, bridge details, and technical resources for each blockchain. Basically everything you need to test and deploy smart contracts or decentralized applications on each chain. For a list of popular Ethereum forums and chat applications click here.
![]() | Ethereum test network configuration and test ETH faucet information |
![]() | Optimistic Ethereum Mainnet and Testnet configuration, bridge details, etc. |
![]() | Polygon network Mainnet and Testnet configuration, faucets for test MATIC tokens, bridge details, etc. |
![]() | Binance Smart Chain Mainnet and Testnet configuration, faucets for test BNB tokens, bridge details, etc. |
![]() | Fanton networt Mainnet and Testnet configuration, faucets for test FTM tokens, bridge details, etc. |
![]() | Kucoin Chain Mainnet and Testnet configuration, faucets for test KCS tokens, bridge details, etc. |
Web3 Software Libraries
You can use the following libraries to interact with an EVM compatible blockchain.
- Python: Web3.py Python library for interacting with Ethereum. Web3.py examples
- Js: web3.js Ethereum JavaScript API
- Java: web3j Web3 Java Ethereum Ðapp API
- PHP: web3.php A php interface for interacting with the Ethereum blockchain and ecosystem.
Nodes
Learn how to run a Geth node. Read getting started with Geth to run an Ethereum node.
Fix a transaction
How to fix a pending transaction stuck on Ethereum or EVM compatible chain