Implement Upkeep using Chainlink Keepers to manage system tasks. Users can not initiate all smart contract actions required to keep a system running. So many application team use centralized services for automation and maintenance tasks. These services are critical infrastructure components to many decentralized applications. Chainlink Keepers enable application development teams to outsource automated centralized tasks to a decentralized platform.
Chainlink Keepers is an off-chain infrastructure layers that interact with smart contracts on the blockchain. It provides developers with access to secure and reliable off-chain smart contract automated computation services. Developers do not need to execute manual tasks, rely on centralized infrastructure services, or implement low level protocol changes to automate on chain events. They have a choice to outsource this work to Chainlink Keepers.
Chainlink Keeper use cases
Most decentralized application teams do not want to manage servers and try to avoid internal automation. Developers can use Chainlink Keepers as a decentralized off-chain computation layer to automate key smart contract functions and custom events. Keepers are similar to a off-chain service, Cron job, or Autosys job that needs to run to maintain an aspect of an application.
Below are several example problems that Chainlink Keepers can help solve. Instead of using a centralized server to manage your system one can use the Chainlink Keeper infrastructure.
- Payment transactions based on new block information
- A liquidation needs to occur due to an under collateralized loan
- An expensive computational transaction needs to occur
- Execute a complex order on a decentralized exchange
- Re invest yields on a defi protocols
- Withdraw commissions every week
- and more
Chainlink Keepers enables off-chain automation of many different types of events. The possibilities are endless.
Decisions to consider for automation tasks
There are many points to consider when determining if you should host or not host automation tasks for an application.
- Reliability is paramount for an application
- The system needs to be easy to use
- Performing all tasks on-chain becomes very expensive over time
- Taking on-chain tasks and moving them off-chain will reduce gas fees
- Do you have safe, secure reliable server to manage these services?
- Do the costs make sense?
- Is cross chain management important?
How do Chainlink Keepers work?
Keepers design consist of three participants:
1. Create an UpKeep compatible smart contract
- Upkeep compatible contracts are smart contracts that need help with maintenance tasks
2. Add the contract to the registry
- Registry is a discovery solution for the above participants to track work
3. Keepers
- Keepers are the actors executing requested Upkeep tasks
- The tasks that Chainlink Keeper perform can, most of the time, be entirely off-chain
- Then perform the Upkeep work
- The blockchain continues to provides security. Only valid transactions can be processes
How to implement a Chainlink Keeper
Lets walk through how to make your contract keeper-compatible, registering it on the Keeper network, and watch the keeper perform tasks. To implement an Upkeep interface you need Link tokens to pay the Keeper and ETH to pay for transactions costs. Request Kovan test tokens using this Link faucet and this page for a list of ETH faucets. Use your favorite exchange to obtain Mainnet tokens.
Keeper compatible smart contract
Implement the KeeperCompatibleInterface and two functions below to make your contract keeper compatible.
- checkUpkeep function is called by the Keeper every block (approximately every 15 seconds). The boolean return value determines if the smart contract needs to be serviced. If the contract requires Upkeep the return bytes are passed to the performUpkeep function.
- performUpkeep function is the Upkeep task that the contract wants servicing on. If checkUpkeep boolean returned true the system calls the performUpkeep function
The interface required to make a contract keeper compatible is on Github at the following Chainlink location:
Example Keeper compatible smart contract
To begin use the sample counter contract below. This contract implements the keeper compatible interface and functions for the keeper. We will use the keeper infrastructure to monitor the blockchain and update the counter in the contract when the block.timestamp is divisible by 7.
First, compile and deploy the contract onto the Ethereum blockchain. All contracts need to be verified on Etherscan for acceptance in the Chainlink keeper network.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
interface KeeperCompatibleInterface {
/**
* @notice checks if the contract requires work to be done.
* @param checkData data passed to the contract when checking for Upkeep.
* @return UpkeepNeeded boolean to indicate whether the keeper should call
* performUpkeep or not.
* @return performData bytes that the keeper should call performUpkeep with,
* if upkeep is needed.
*/
function checkUpkeep(bytes calldata checkData) external returns (bool upkeepNeeded,bytes memory performData);
/**
* @notice Performs work on the contract. Executed by the keepers, via the registry.
* @param performData is the data which was passed back from the checkData
* simulation.
*/
function performUpkeep(bytes calldata performData) external;
}
contract counterContract is KeeperCompatibleInterface {
//counter variable
uint public counter;
//function to test your counter
function increaseCounter() public {
counter += 1;
}
//used to view the current block.timestamp
function timeStamp() public view returns (uint) {
return block.timestamp;
}
// check to see if the block.timestamp is divisible by 7
// if true call the performUpkeep function
function checkUpkeep(bytes calldata /* checkData */) external override returns (bool upkeepNeeded, bytes memory /* performData */) {
upkeepNeeded = (block.timestamp % 7 == 0);
}
//if the block.timestamp is divisible by 7 increase the counter (function checkUpkeep above)
//keeper will perform update
function performUpkeep(bytes calldata /* performData */) external override {
counter = counter + 1;
}
}
Try it in Remix
Register on the Keeper network
Second, register your contract on the Keeper Network. Go to the Chainlink Keepers portal to register your contract for monitored maintenance. Connect your wallet to the Chainlink website and select register new Upkeep.
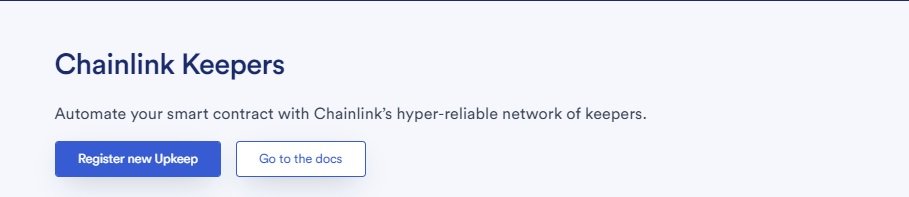
Next complete the request form so the Keeper environment knows what contract to monitor. The information you provide will be publicly visible on the blockchain. Chainlink encrypts email address information. Make sure you have Link tokens to fund your Upkeep.
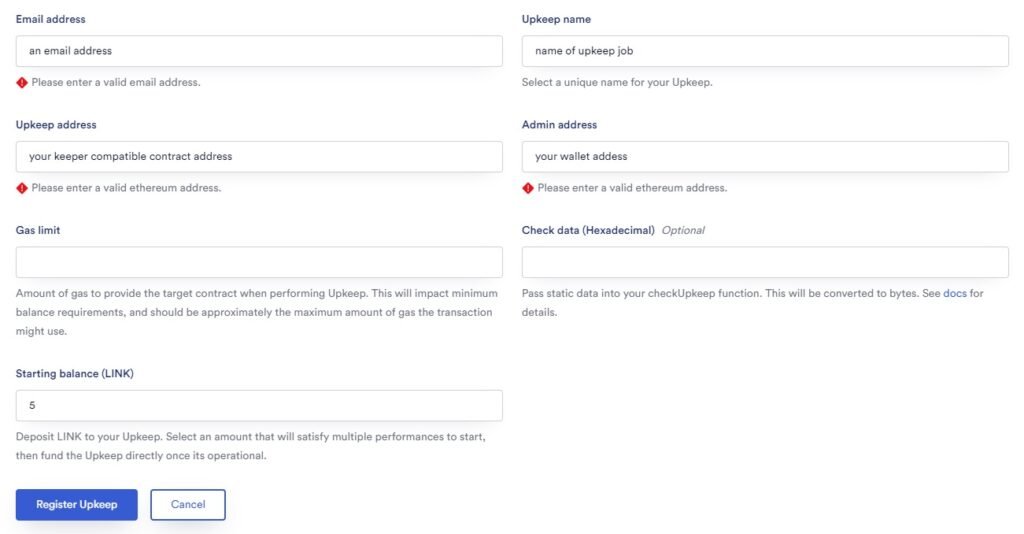
After completing the form press register Upkeep and confirm the transaction in MetaMask. Chainlink Keeper requests are automatically approved and you should be up and running in a matter of minutes.
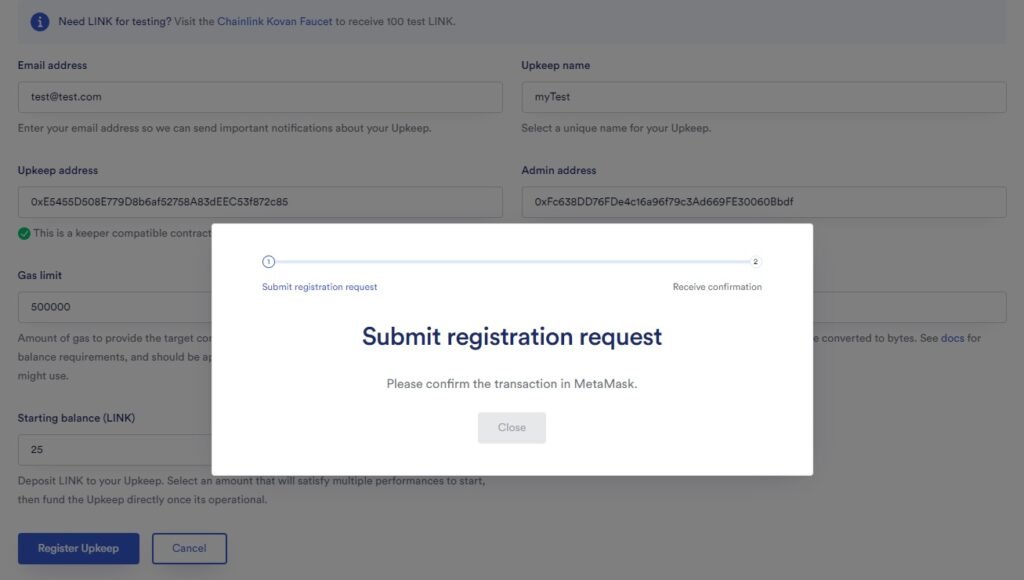
Approve MetaMask transaction to process Upkeep request. Make sure the environment is correct.
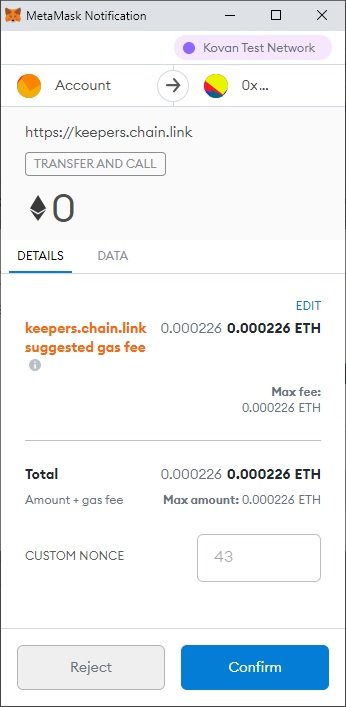
You will receive a confirmation once the transaction is complete.
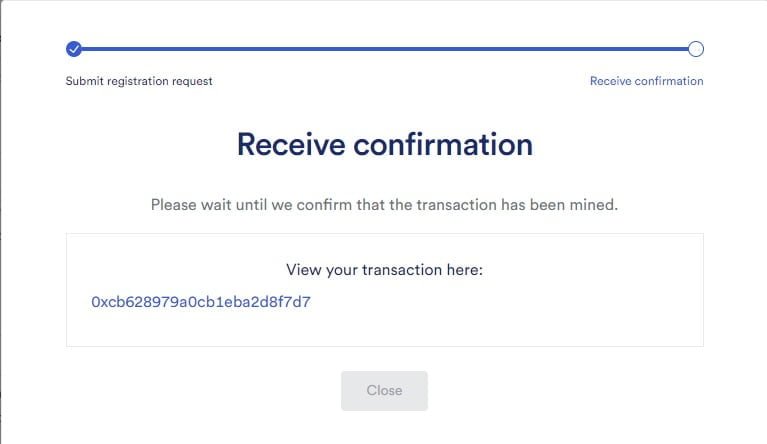
After the transaction is complete and the window above is closed you will be directed to the home page of the Chainlink Keepers app. On this page you can view your scheduled jobs and their details:
- Status of Keeper job
- Link balance
- Minimum Link balance
- Date added to the system
- Gas limit
- Add funds request
- Cancel Upkeep request
- History of Upkeep work
- etc.
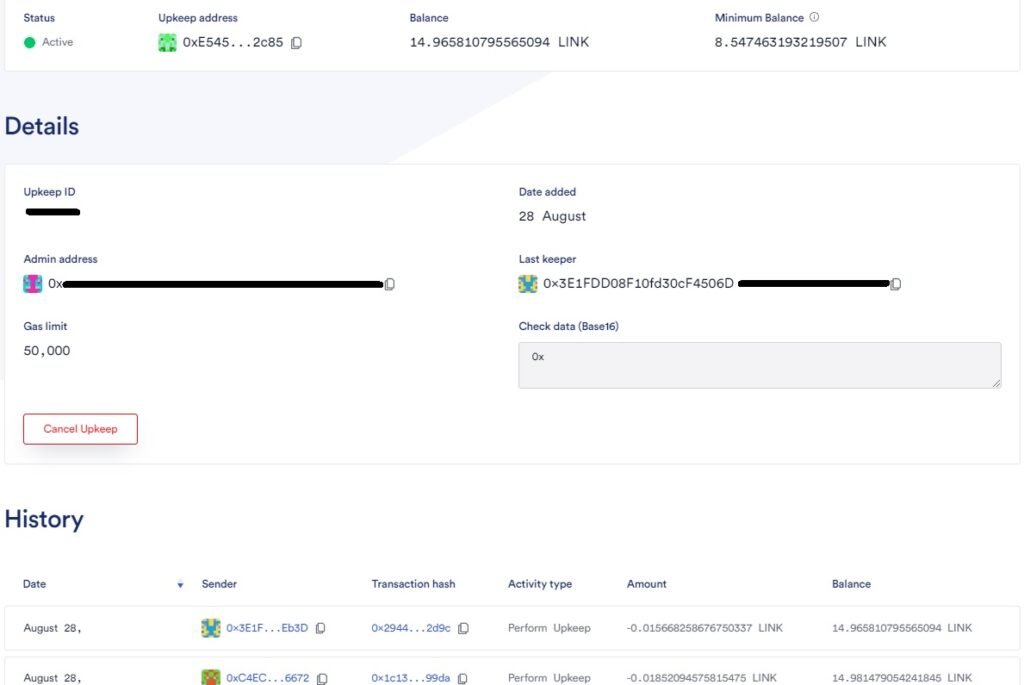
Verify Upkeep
Finally, after waiting about 5-10 minutes, navigate to your contract and check if the counter changed. The contract instructed the keeper to update the counter when a condition is met. If the counter changed the keeper is doing its job.

Now navigate back to your Chainlink Keepers app web page. After you refresh the page you can see the history of work generated by the keeper. According to the Kovan test data below the keeper charged .015686 link per transaction executed.

Paying for Upkeep and adding funds
- Your Chainlink balance is reduced each time a Keeper executes your performUpkeep function
- There is no cost for checkUpkeep calls
- If you want to automate adding funds call the addfunds() function on the keeperRegistry contract
- Anyone (not just the Upkeep owner) can call the addfunds() function
- To withdraw funds first cancel the Upkeep job then withdraw funds
To ensure the Chainlink Keepers are compensated for their services, there is an expected minimum balance on each Upkeep. If your funds drop below the specified amount, the Upkeep will not be performed. If your balance runs low you can press the Add funds button on the Chainlink Keepers app web page to add Chainlink tokens to your job.

Cancel you Upkeep job
If you want to cancel your upkeep job press the cancel button on the Chainlink Keepers app web page. This will require you to sign a message to confirm the cancelation.

With Chainlink Keepers you can create a decentralized hybrid application. As systems become more decentralized innovation will grow exponentially. Once the ecosystem gets comfortable using Keepers the use will be widespread.
Chainlink Keeper best practices
For information on best practices visit the Chainlink Keeper best practices documentation. They review design patterns, modifiers and configuration to use in your contract.
Resources
Blockchain Networks
Below is a list of EVM compatible Mainnet and Testnet blockchain networks. Each link contains network configuration, links to multiple faucets for test ETH and tokens, bridge details, and technical resources for each blockchain. Basically everything you need to test and deploy smart contracts or decentralized applications on each chain. For a list of popular Ethereum forums and chat applications click here.
![]() | Ethereum test network configuration and test ETH faucet information |
![]() | Optimistic Ethereum Mainnet and Testnet configuration, bridge details, etc. |
![]() | Polygon network Mainnet and Testnet configuration, faucets for test MATIC tokens, bridge details, etc. |
![]() | Binance Smart Chain Mainnet and Testnet configuration, faucets for test BNB tokens, bridge details, etc. |
![]() | Fanton networt Mainnet and Testnet configuration, faucets for test FTM tokens, bridge details, etc. |
![]() | Kucoin Chain Mainnet and Testnet configuration, faucets for test KCS tokens, bridge details, etc. |
Web3 Software Libraries
You can use the following libraries to interact with an EVM compatible blockchain.
- Python: Web3.py Python library for interacting with Ethereum. Web3.py examples
- Js: web3.js Ethereum JavaScript API
- Java: web3j Web3 Java Ethereum Ðapp API
- PHP: web3.php A php interface for interacting with the Ethereum blockchain and ecosystem.
Nodes
Learn how to run a Geth node. Read getting started with Geth to run an Ethereum node.
Fix a transaction
How to fix a pending transaction stuck on Ethereum or EVM compatible chain