If you are getting started with Solidity development we recommend you begin by reading the tutorials outline below. They will help you understand important core concepts in Ethereum smart contract development.
Solidity Integrated Development Environments (IDE) and tools
To begin set up your development environment and select an IDE. Our recommendation is to use Remix. Then become familiar with the other development tools using the link below. These tools will help you navigate the environment and trouble shoot issues. Make sure you know how to connect your IDE to the test environments (links below).
Introduction to Solidity with Examples
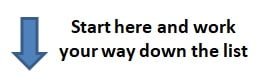
- Data types
- Constructors
- Variables
- Functions
- Visibility
- Pure and View
- Function modifiers
- Gas
- Events
- Error handling
- Fallback function
- For Loop
- Array
- Mapping
- Enum
- Struct
- Payable modifier
- Import into a contract
- Contract cleanup
- Memory vs storage
- Inheritance
- If statements
- Libraries
- Interface
- New keyword
- Dynamic array
- Try catch
- Modules
- Account abstraction
- mstore and mload
- Memory Impacts the Cost of Ethereum Gas
Sample Solidity smart contracts
After reading about each of the Solidity core concepts above read the sample contracts below. Rewrite the contracts in Remix or your favorite IDE and deploy them to the test network. Practicing syntax and concepts using real examples is the best way to learn.
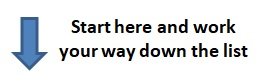
- Sample contract
- Address book
- Hotel contract or vending machine
- Time lock
- Simple ERC20 token contract
- Shared wallet
- Trustless token swap
- Eth game deposit and win
- Delegate call to upgrade a contract
- Covered call option
- Uniswap clone
- Multi signature wallet
- Chain link oracle
- Chain link price feeds on Binance Smart Chain
- Yield farm
- How to create an ERC721 Solidity smart contract
- How to swap tokens on Uniswap using a smart contract
- Create a Defi Bank that pays interest (Yield Farm)
- How to sign & verify an Ethereum message off chain (Part 1)
- How to create a payment channel on Ethereum (Part 2)
- Lock a token so it can not be transferred
- Auto send a percent of tokens to another address
- Roulette Game
- Flash loan arbitrage on Uniswap and SushiSwap
- Use a signature to generate a public key
- How to fork the SafeMoon token smart contract
- Multiple ways to upgrade a Solidity smart contract
- Compound Finance liquidation smart contract
- Implement Upkeep using Chainlink Keepers
- How to create and deploy an NFT collection
- Create a crypto faucet using a Solidity smart contract
- ERC721 contract that supports sales royalties
- Dynamic NFTs on Ethereum
- What is an ERC-1404 token contract
- Vaults and the ERC-4626 token contract
- Subscription payments
- How to create a DAO
- Implement an escrow service
- Create an ERC-5573 multi asset token contract
- ERC-1155 contracts that support multiple tokens
- Understanding Ethereum Contract Type 4337
Resources
Blockchain Networks
Getting started with Solidity? Below is a list of EVM compatible Mainnet and Testnet blockchain networks. Each link contains network configuration, links to multiple faucets for test ETH and tokens, bridge details, and technical resources for each blockchain. Basically everything you need to test and deploy Solidity smart contracts or decentralized applications on each chain. For a list of popular Ethereum forums and chat applications click here.
![]() | Ethereum test network configuration and test ETH faucet information |
![]() | Optimistic Ethereum Mainnet and Testnet configuration, bridge details, etc. |
![]() | Polygon network Mainnet and Testnet configuration, faucets for test MATIC tokens, bridge details, etc. |
![]() | Binance Smart Chain Mainnet and Testnet configuration, faucets for test BNB tokens, bridge details, etc. |
![]() | Fanton networt Mainnet and Testnet configuration, faucets for test FTM tokens, bridge details, etc. |
![]() | Kucoin Chain Mainnet and Testnet configuration, faucets for test KCS tokens, bridge details, etc. |
Web3 Software Libraries
You can use the following libraries to interact with an EVM compatible blockchain.
- Python: Web3.py A Python library for interacting with Ethereum. Web3.py examples
- Js: web3.js Ethereum JavaScript API
- Java: web3j Web3 Java Ethereum Ðapp API
- PHP: web3.php A php interface for interacting with the Ethereum blockchain and ecosystem.
Nodes
Learn how to run a Geth node. Read getting started with Geth to run an Ethereum node
Fix a transaction
How to fix a pending transaction stuck on Ethereum or EVM compatible chain
Additional development resources and projects
Search here for additional development projects and information
Getting Started with Solidity should be easy
The information above is for educational and entertainment purposes only